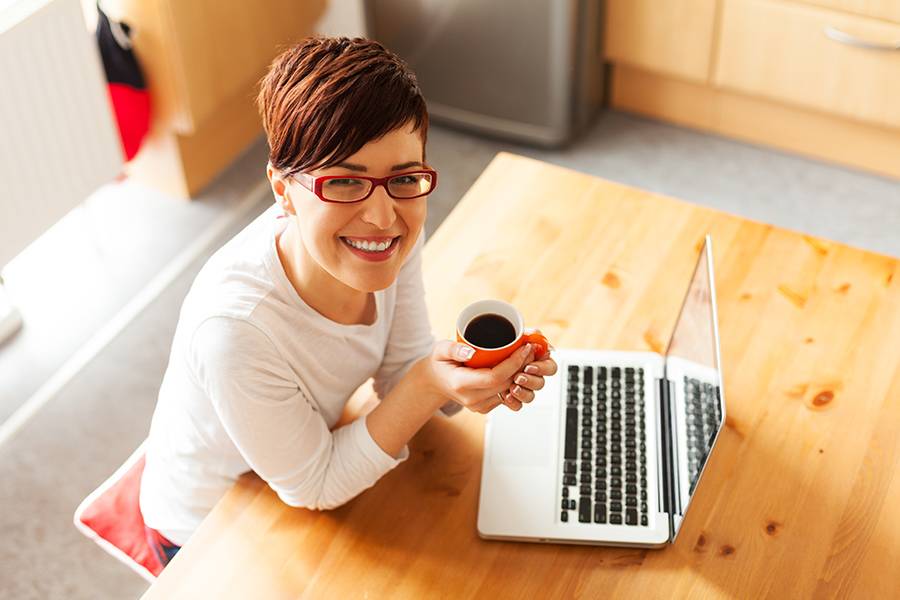
Course Description: Embark on your journey into iOS app development with our comprehensive “Swift Programming for Beginners” course. Designed for individuals with little to no programming experience, this course provides a solid foundation in Swift, Apple’s powerful and intuitive programming language for iOS, macOS, watchOS, and tvOS development. Through a combination of theory, practical examples, and hands-on exercises, you’ll learn the fundamentals of Swift programming and gain the skills needed to start building your own iOS apps.
Course Curriculum:
- Introduction to Swift
- Overview of Swift programming language
- Setting up Xcode IDE for Swift development
- Exploring Swift playgrounds for interactive coding
- Swift Basics
- Syntax and structure of Swift code
- Data types and variables
- Constants and control flow statements: if, else, switch, loops
- Functions and Methods
- Declaring and calling functions in Swift
- Parameters and return values
- Working with methods and method overloading
- Collections and Data Structures
- Arrays, dictionaries, sets, and tuples
- Accessing and manipulating collection elements
- Working with optional values in Swift
- Object-Oriented Programming in Swift
- Classes, objects, and instances
- Properties and methods in classes
- Inheritance, polymorphism, and encapsulation
- Error Handling
- Understanding errors and exceptions in Swift
- Handling errors using do-catch blocks
- Propagating errors using throw and try
- Introduction to iOS App Development
- Overview of iOS app architecture
- Understanding the Model-View-Controller (MVC) design pattern
- Creating user interfaces with Interface Builder and Storyboards
- Introduction to UIKit Framework
- Overview of UIKit framework for iOS development
- Creating UI components: labels, buttons, text fields, etc.
- Handling user interactions and events
- Working with Views and View Controllers
- Understanding views and view hierarchies
- Creating custom views and view controllers
- Navigating between view controllers using segues
- Introduction to Networking and Data Persistence
- Making network requests using URLSession
- Parsing JSON data using Codable
- Saving and retrieving data using UserDefaults
- Debugging and Testing
- Debugging techniques in Xcode
- Writing and running unit tests in Swift
- Best practices for debugging and testing iOS apps
- Publishing Your App
- App distribution options: App Store vs TestFlight
- Preparing your app for submission to the App Store
- Tips for app marketing and promotion
Prerequisites: No prior programming experience is required. Basic familiarity with computers and software applications is recommended.
Duration: This course is self-paced, with approximately XX hours of content.
Certification: Upon successful completion of the course, you’ll receive a certificate of completion from First Paisa. Additionally, you’ll be well-equipped to start building your own iOS apps using Swift.
2 thoughts on “Swift programming for beginners”
- 43 hours on-demand video
- 81 articles
- 19 downloadable resources
- 4 coding exercises
- Full lifetime access
- Access on mobile and TV
- Certificate of Completion
This course is amazing, it is extremely thorough and in depth! I am not finished with the course, but just the excel exercises alone are more than worth the price of the course! A must take course! Thank you!
Great starting point for learning Swift. If you have never programmed, or never used Swift it is a great place to start.